Integrate a twitter timeline in your android application.
Quick tutorial for integrating Twitter timeline into Android app using WebView - 30-minute social feature implementation for BresTram app with embedded Twitter widget and transparent background.
Hey all,
Note: The solution mentioned in this article is not functional any more after all the amazing changes that Mr. Elno brought to Twitter (😖, I mean X 🤢). There is no way to embed your timeline like this any more without paying. 🤷♂️
This week-end, I worked on integrating a social feature in BresTram.
I needed a way to let the users know when the bibus servers have a problem, and also let users send messages to each others if needed.
Finally, I didn't want to spend too much time developing it. I have more pressing features to implement, and the social integration is more of a "test".
I decided to go with Twitter. The message will generally be short (work on the rails, problems with the tram, ...), and everybody should be able to see them.
I started looking at the Streaming API, but then realized there was a much simpler way to do it : Simply integrate a web timeline into my app.
I started by creating a dedicated filter on the twitter account of BresTram.
This gives me two lines of code to integrate into the website of my choice :
<a class="twitter-timeline" href="http://twitter.com/search?q=%23BresTram" data-widget-id="394415351972114432">Tweets about "#BresTram"</a>
<script>!function(d,s,id){var js,fjs=d.getElementsByTagName(s)[0],p=/^http:/.test(d.location)?'http':'https';if(!d.getElementById(id)){js=d.createElement(s);js.id=id;js.src=p+"://platform.twitter.com/widgets.js";fjs.parentNode.insertBefore(js,fjs);}}(document,"script","twitter-wjs");</script>
Then, I simply created a new Activity in my Application, that contains a webview.
package fr.lengrand.brestram;
import android.os.Bundle;
import android.support.v7.app.ActionBarActivity;
import android.view.Menu;
import android.view.MenuItem;
import android.webkit.WebView;
public class MainActivity extends ActionBarActivity {
public static final String TAG = "TimeLineActivity";
private static final String baseURl = "http://twitter.com";
private static final String widgetInfo = "<a class=\"twitter-timeline\" href=\"http://twitter.com/search?q=%23BresTram\" data-widget-id=\"394415351972114432\">Tweets about \"#BresTram\"</a> " +
"<script>!function(d,s,id){var js,fjs=d.getElementsByTagName(s)[0],p=/^http:/.test(d.location)?'http':'https';if(!d.getElementById(id)){js=d.createElement(s);js.id=id;js.src=p+\"://platform.twitter.com/widgets.js\";fjs.parentNode.insertBefore(js,fjs);}}(document,\"script\",\"twitter-wjs\");</script>";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
load_background_color();
WebView webView = (WebView) findViewById(R.id.timeline_webview);
webView.getSettings().setDomStorageEnabled(true);
webView.getSettings().setJavaScriptEnabled(true);
webView.loadDataWithBaseURL(baseURl, widgetInfo, "text/html", "UTF-8", null);
}
private void load_background_color() {
WebView webView = (WebView) findViewById(R.id.timeline_webview);
//webView.setBackgroundColor(getResources().getColor(R.color.twitter_dark));
webView.setBackgroundColor(0); // transparent
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
return true;
}
}
The background color method is needed to avoid the ugly white background behind the timeline. I use a transparent background in this case.
It is not possible to set the background directly in the xml layout for webviews; you will have to do it in the activity directly.
And here is the layout file :
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".TimeLineActivity" >
<WebView
android:id="@+id/timeline_webview"
android:layout_width="fill_parent"
android:layout_height="fill_parent"/>
</RelativeLayout>
I used fill_parent because I wanted to give as much space as possible to the Webview.
The last thing you want to do is make sure you are allowing your app to make web calls. For that, add this line in your Android Manifest.
<uses-permission android:name="android.permission.INTERNET" />
The result is far from perfect, but it's not that bad for roughly 30 minutes of work :).
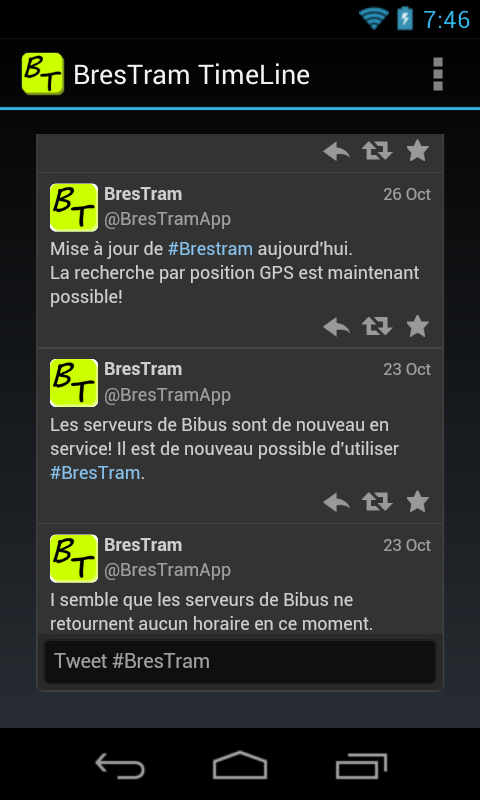
On top of that, It directly allows my users to post tweets from the application (using the timeline), and will let everybody know about the BresTram twitter account.
Hopefully, this will help me get some useful feedback.
Now, back to more complex features :).
Oh, and if you leave near Brest, check out BresTram!
P.S: This is not exactly how the actual source code look like. I changed several things to highlight the essentials in this post.
EDIT : Edited after a request on 28th Feb. 2015.